Pretty Print JSON in Linux Terminal
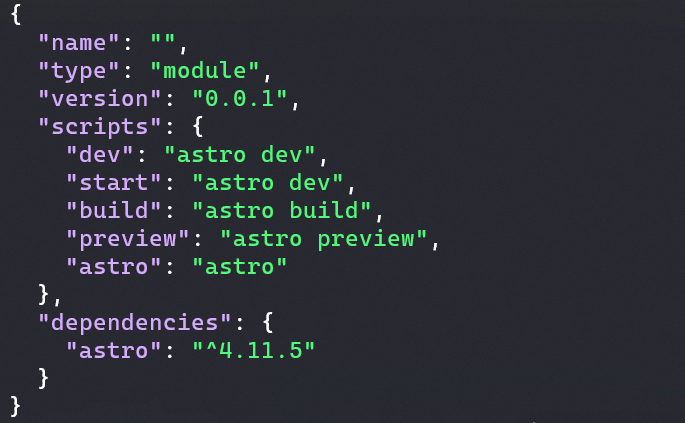
Table of Contents
About
JSON (JavaScript Object Notation) is a widely used data format for exchanging information between web clients and servers. However, JSON data is often densely packed, making it difficult to read when viewing it directly in the terminal. Fortunately, Linux offers several tools to help pretty-print JSON files, making them easier to read and analyze. In this post, we’ll explore various methods for formatting JSON in the Linux terminal, including jq
, Python’s built-in JSON tool, and a custom Bash script for environments where installing new tools is not possible.
Using jq
Installation
If jq
is not installed on your system, you can usually get it via your package manager:
Usage
jq
is a powerful command-line JSON processor. It’s a versatile tool that can be used not only for pretty-printing JSON data but also for parsing, filtering, and transforming it.
Here’s how you can use jq to pretty-print a JSON file:
The .
in the command represents the identity filter, which outputs the JSON as-is but formatted nicely.
Another, more common, way to use jq
is to pipe the results of commands or file output to jq
and it will pretty-print the JSON data in the standard output:
Using Python’s Built-in JSON Tool
Installation
If Python is not installed in your Linux distribution, you can usually get it via your package manager:
Usage
Python’s standard library includes a module called json.tool
, which can be used as a simple way to pretty-print JSON. This is particularly handy because Python is pre-installed on most Linux distributions. Here’s the command to pretty-print a JSON file using Python:
The command reads the JSON file, formats it, and prints it to the standard output.
Similary, we can pipe the results of commands or file output to jq
and it will pretty-print the JSON data in the standard output:
Using custom Bash script
In certain environments, such as systems or Docker containers without internet access, installing new tools may not be feasible. In such cases, a custom Bash script can be used to format JSON files. Here is a simple script that accomplishes this:
How It Works
- Input: The script takes a JSON file as input.
- Indentation: It increases the indentation level after encountering an opening brace
{
or bracket[
, and decreases it after a closing brace}
or bracket]
. - New Lines: It inserts new lines and spaces to align braces and brackets properly.
Installation
We can save the above function to our ~/.bashrc
script in order to have the jsonf
command available at login. After that we can source the file for our chances to be applied:
Usage
We can pretty-print a JSON file using the above bash function with:
The function reads the JSON file, formats it, and prints it to the standard output.
Similary, we can pipe the results of commands or file output to jsonf
and it will pretty-print the JSON data in the standard output: